R Provider Tutorial
Referencing the provider
In order to use the R provider, you need to reference the RDotNet.dll
library
(which is a .NET connector for R) and the RProvider.dll
itself. For this tutorial,
we use open
to reference a number of packages including stats
, tseries
and zoo
:
1: 2: 3: 4: 5: 6: 7: 8: 9: 10: 11: 12: 13: 14: 15: 16: |
|
If either of the namespaces above are unrecognized, you need to install the package in R
using install.packages("stats")
.
Obtaining data
In this tutorial, we use F# Data to access stock prices from the Yahoo Finance portal. For more information, see the documentation for the CSV type provider.
The following snippet uses the CSV type provider to generate a type Stocks
that can be
used for parsing CSV data from Yahoo. Then it defines a function getStockPrices
that returns
array with prices for the specified stock and a specified number of days:
1: 2: 3: 4: 5: 6: 7: 8: 9: 10: 11: 12: 13: 14: |
|
Calling R functions
Now, we're ready to call R functions using the type provider. The following snippet takes
msftOpens
, calculates logarithm of the values using R.log
and then calculates the
differences of the resulting vector using R.diff
:
1: 2: |
|
If you want to see the resulting values, you can call msft.AsVector()
in F# Interactive.
Next, we use the acf
function to display the atuo-correlation and call adf_test
to
see if the msft
returns are stationary/non-unit root:
1: 2: |
|
After running the first snippet, a window similar to the following should appear (note that it might not appear as a top-most window).
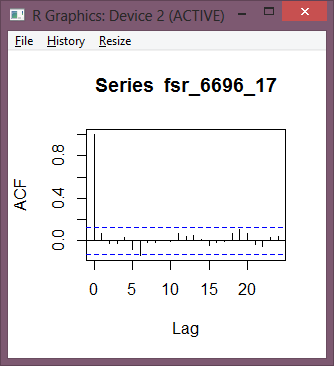
Finally, we can obtain data for multiple different indicators and use the R.pairs
function
to produce a matrix of scatter plots:
1: 2: 3: 4: 5: 6: 7: 8: 9: 10: 11: |
|
As a result, you should see a window showing results similar to these:
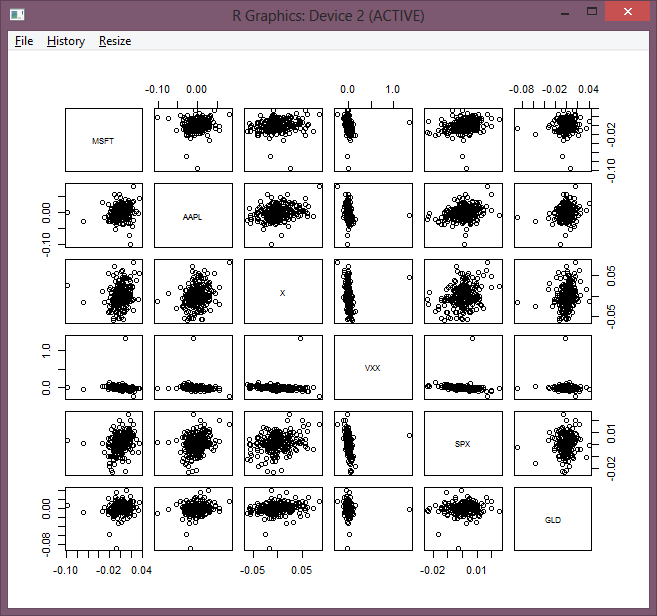
Full name: Tutorial.Stocks
Full name: FSharp.Data.CsvProvider
<summary>Typed representation of a CSV file.</summary>
<param name='Sample'>Location of a CSV sample file or a string containing a sample CSV document.</param>
<param name='Separators'>Column delimiter(s). Defaults to `,`.</param>
<param name='InferRows'>Number of rows to use for inference. Defaults to `1000`. If this is zero, all rows are used.</param>
<param name='Schema'>Optional column types, in a comma separated list. Valid types are `int`, `int64`, `bool`, `float`, `decimal`, `date`, `guid`, `string`, `int?`, `int64?`, `bool?`, `float?`, `decimal?`, `date?`, `guid?`, `int option`, `int64 option`, `bool option`, `float option`, `decimal option`, `date option`, `guid option` and `string option`.
You can also specify a unit and the name of the column like this: `Name (type<unit>)`, or you can override only the name. If you don't want to specify all the columns, you can reference the columns by name like this: `ColumnName=type`.</param>
<param name='HasHeaders'>Whether the sample contains the names of the columns as its first line.</param>
<param name='IgnoreErrors'>Whether to ignore rows that have the wrong number of columns or which can't be parsed using the inferred or specified schema. Otherwise an exception is thrown when these rows are encountered.</param>
<param name='SkipRows'>SKips the first n rows of the CSV file.</param>
<param name='AssumeMissingValues'>When set to true, the type provider will assume all columns can have missing values, even if in the provided sample all values are present. Defaults to false.</param>
<param name='PreferOptionals'>When set to true, inference will prefer to use the option type instead of nullable types, `double.NaN` or `""` for missing values. Defaults to false.</param>
<param name='Quote'>The quotation mark (for surrounding values containing the delimiter). Defaults to `"`.</param>
<param name='MissingValues'>The set of strings recogized as missing values. Defaults to `NaN,NA,N/A,#N/A,:,-,TBA,TBD`.</param>
<param name='CacheRows'>Whether the rows should be caches so they can be iterated multiple times. Defaults to true. Disable for large datasets.</param>
<param name='Culture'>The culture used for parsing numbers and dates. Defaults to the invariant culture.</param>
<param name='Encoding'>The encoding used to read the sample. You can specify either the character set name or the codepage number. Defaults to UTF8 for files, and to ISO-8859-1 the for HTTP requests, unless `charset` is specified in the `Content-Type` response header.</param>
<param name='ResolutionFolder'>A directory that is used when resolving relative file references (at design time and in hosted execution).</param>
<param name='EmbeddedResource'>When specified, the type provider first attempts to load the sample from the specified resource
(e.g. 'MyCompany.MyAssembly, resource_name.csv'). This is useful when exposing types generated by the type provider.</param>
Full name: Tutorial.getStockPrices
Returns prices of a given stock for a specified number
of days (starting from the most recent)
val float : value:'T -> float (requires member op_Explicit)
Full name: Microsoft.FSharp.Core.Operators.float
--------------------
type float = Double
Full name: Microsoft.FSharp.Core.float
--------------------
type float<'Measure> = float
Full name: Microsoft.FSharp.Core.float<_>
member Clone : unit -> obj
member CopyTo : array:Array * index:int -> unit + 1 overload
member GetEnumerator : unit -> IEnumerator
member GetLength : dimension:int -> int
member GetLongLength : dimension:int -> int64
member GetLowerBound : dimension:int -> int
member GetUpperBound : dimension:int -> int
member GetValue : params indices:int[] -> obj + 7 overloads
member Initialize : unit -> unit
member IsFixedSize : bool
...
Full name: System.Array
Full name: Microsoft.FSharp.Collections.Array.rev
Full name: Tutorial.msftOpens
Get opening prices for MSFT for the last 255 days
Full name: Tutorial.msft
static member ! : ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member != : ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member !_hexmode : ?a: obj -> SymbolicExpression + 1 overload
static member !_octmode : ?a: obj -> SymbolicExpression + 1 overload
static member $ : ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member $<- : ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member $<-_data_frame : ?x: obj * ?name: obj * ?value: obj -> SymbolicExpression + 1 overload
static member $_DLLInfo : ?x: obj * ?name: obj -> SymbolicExpression + 1 overload
static member $_data_frame : ?x: obj * ?name: obj -> SymbolicExpression + 1 overload
static member $_package__version : ?x: obj * ?name: obj -> SymbolicExpression + 1 overload
...
Full name: RProvider.R
Base R functions.
R.log(?paramArray: obj []) : SymbolicExpression
Logarithms and Exponentials
R.diff(?x: obj, ?___: obj, ?paramArray: obj []) : SymbolicExpression
Lagged Differences
Full name: Tutorial.a
static member AIC : ?object: obj * ?___: obj * ?k: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member ARMAacf : ?ar: obj * ?ma: obj * ?lag_max: obj * ?pacf: obj -> SymbolicExpression + 1 overload
static member ARMAtoMA : ?ar: obj * ?ma: obj * ?lag_max: obj -> SymbolicExpression + 1 overload
static member BIC : ?object: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member Box_test : ?x: obj * ?lag: obj * ?type: obj * ?fitdf: obj -> SymbolicExpression + 1 overload
static member C : ?object: obj * ?contr: obj * ?how_many: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member D : ?expr: obj * ?name: obj -> SymbolicExpression + 1 overload
static member Gamma : ?link: obj -> SymbolicExpression + 1 overload
static member HoltWinters : ?x: obj * ?alpha: obj * ?beta: obj * ?gamma: obj * ?seasonal: obj * ?start_periods: obj * ?l_start: obj * ?b_start: obj * ?s_start: obj * ?optim_start: obj * ?optim_control: obj -> SymbolicExpression + 1 overload
static member IQR : ?x: obj * ?na_rm: obj * ?type: obj -> SymbolicExpression + 1 overload
...
Full name: RProvider.stats.R
R statistical functions.
R.acf(?x: obj, ?lag_max: obj, ?type: obj, ?plot: obj, ?na_action: obj, ?demean: obj, ?___: obj, ?paramArray: obj []) : SymbolicExpression
Auto- and Cross- Covariance and -Correlation Function Estimation
Full name: Tutorial.adf
static member MATCH : ?x: obj * ?table: obj * ?nomatch: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member MATCH_default : ?x: obj * ?table: obj * ?nomatch: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member MATCH_times : ?x: obj * ?table: obj * ?nomatch: obj * ?units: obj * ?eps: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member ORDER : ?x: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member ORDER_default : ?x: obj * ?___: obj * ?na_last: obj * ?decreasing: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member Sys_yearmon : ?NULL: obj -> SymbolicExpression + 1 overload
static member Sys_yearqtr : ?NULL: obj -> SymbolicExpression + 1 overload
static member as_Date : ?x: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member as_Date_numeric : ?x: obj * ?origin: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member as_Date_ts : ?x: obj * ?offset: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
...
Full name: RProvider.zoo.R
An S3 class with methods for totally ordered indexed
observations. It is particularly aimed at irregular time series
of numeric vectors/matrices and factors. zoo's key design goals
are independence of a particular index/date/time class and
consistency with ts and base R by providing methods to extend
standard generics.
Full name: Tutorial.tickers
Full name: Tutorial.data
Full name: Microsoft.FSharp.Core.ExtraTopLevelOperators.printfn
Full name: Tutorial.df
R.data_frame(?___: obj, ?row_names: obj, ?check_rows: obj, ?check_names: obj, ?stringsAsFactors: obj, ?paramArray: obj []) : SymbolicExpression
Data Frames
Full name: RProvider.Helpers.namedParams
static member Axis : ?x: obj * ?at: obj * ?___: obj * ?side: obj * ?labels: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member abline : ?a: obj * ?b: obj * ?h: obj * ?v: obj * ?reg: obj * ?coef: obj * ?untf: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member arrows : ?x0: obj * ?y0: obj * ?x1: obj * ?y1: obj * ?length: obj * ?angle: obj * ?code: obj * ?col: obj * ?lty: obj * ?lwd: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member assocplot : ?x: obj * ?col: obj * ?space: obj * ?main: obj * ?xlab: obj * ?ylab: obj -> SymbolicExpression + 1 overload
static member axTicks : ?side: obj * ?axp: obj * ?usr: obj * ?log: obj * ?nintLog: obj -> SymbolicExpression + 1 overload
static member axis : ?side: obj * ?at: obj * ?labels: obj * ?tick: obj * ?line: obj * ?pos: obj * ?outer: obj * ?font: obj * ?lty: obj * ?lwd: obj * ?lwd_ticks: obj * ?col: obj * ?col_ticks: obj * ?hadj: obj * ?padj: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member axis_Date : ?side: obj * ?x: obj * ?at: obj * ?format: obj * ?labels: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member axis_POSIXct : ?side: obj * ?x: obj * ?at: obj * ?format: obj * ?labels: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member barplot : ?height: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
static member barplot_default : ?height: obj * ?width: obj * ?space: obj * ?names_arg: obj * ?legend_text: obj * ?beside: obj * ?horiz: obj * ?density: obj * ?angle: obj * ?col: obj * ?border: obj * ?main: obj * ?sub: obj * ?xlab: obj * ?ylab: obj * ?xlim: obj * ?ylim: obj * ?xpd: obj * ?log: obj * ?axes: obj * ?axisnames: obj * ?cex_axis: obj * ?cex_names: obj * ?inside: obj * ?plot: obj * ?axis_lty: obj * ?offset: obj * ?add: obj * ?args_legend: obj * ?___: obj * ?paramArray: obj [] -> SymbolicExpression + 1 overload
...
Full name: RProvider.graphics.R
R functions for base graphics.
R.pairs(?x: obj, ?___: obj, ?paramArray: obj []) : SymbolicExpression
Scatterplot Matrices